Thusly
Programming Language
Developer
Elle J
Category
Language
Live Site
Source Code
Release Year
2023
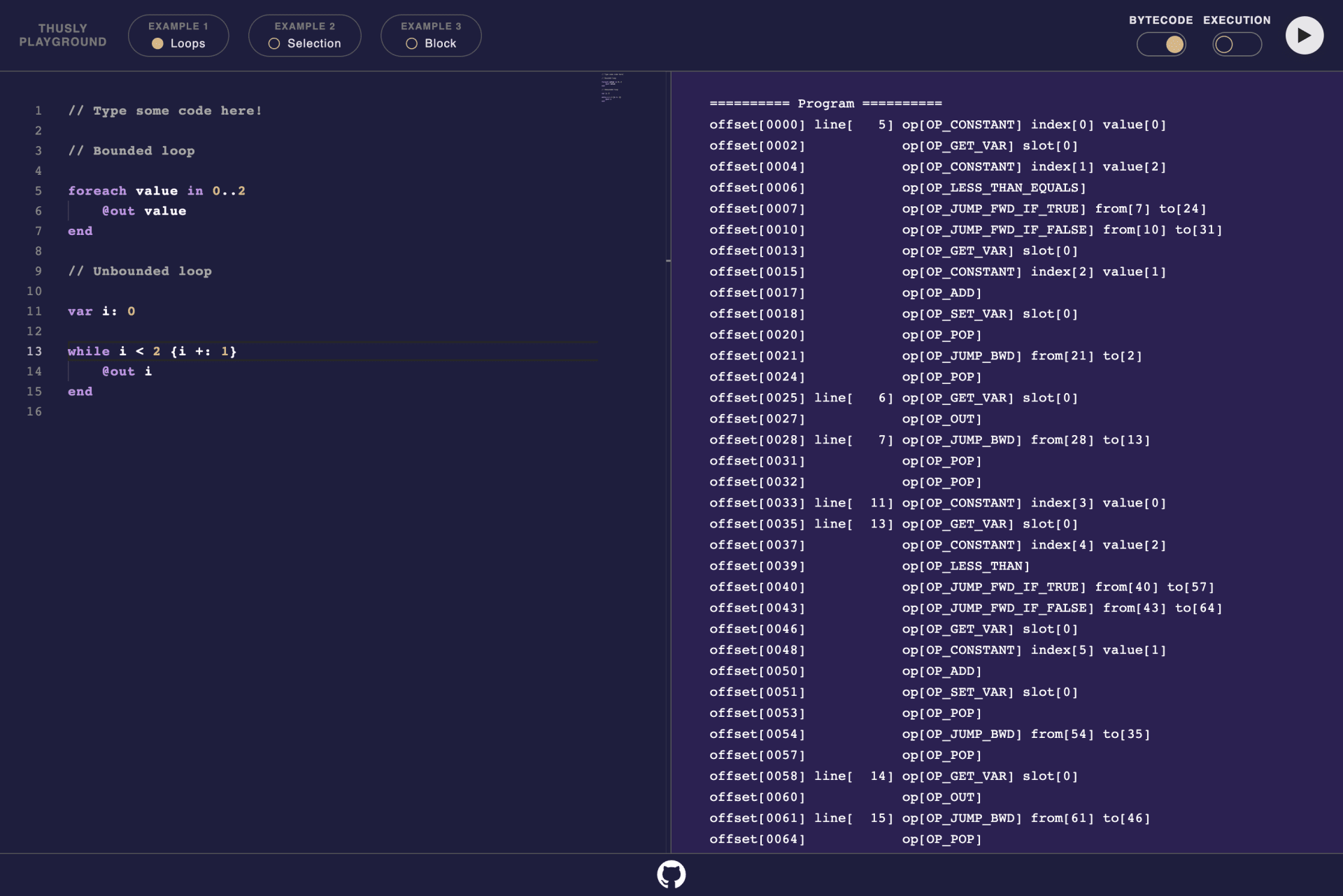
Thusly is an open-source general-purpose programming language originally developed for personally deepening my knowledge of compilers, virtual machines, and the C language. The implementation is a one-pass compiler and a stack-based virtual machine.
👉️ Thusly is currently being developed. See Milestones for implemented features.
Table of Contents
Playground
Try out Thusly in the interactive playground.
Language
Characteristics
- General-purpose
- Interpreted
- Dynamically typed
- Imperative
- Garbage collected
Syntax
The following is a subset of the language:
Variables, Data Types, and Literals
const id: 100
var valid: true
var enabled: false
var nickname: none
nickname: "Alex"
Selection
var age: 30
if age in 25..35
@out("You are young")
end
var timeLeft: 120
var cancelled: false
if timeLeft = 0 or cancelled
@out("Done")
elseif timeLeft < 60
@out("Almost done")
else
@out("Keep going")
end
Loop
foreach value in 0..10
@out(value)
end
var a: 0
var b: 10
var c: 2
foreach value in a..b step c
@out(value)
end
var i: 0
while i < 10 {i +: 1}
@out(i)
end
Whitespace is semantically insignificant except for newline characters on non-blank lines.
Milestones
Milestone 1: Support arithmetic, comparison, and equality operations
- ✅ The terminals in the initial grammar can be identified from user input via a multi-line file or single-line REPL input and then tokenized.
- ✅ Arithmetic expressions using
number
(double) can be evaluated.- ✅ Addition (
+
) - ✅ Subtraction (
-
) - ✅ Multiplication (
*
) - ✅ Division (
/
) - ✅ Modulo (
mod
) - ✅ Unary negation (
-
) - ✅ Precedence altering (
()
)
- ✅ Addition (
- ✅ Comparison expressions using
number
can be evaluated.- ✅ Greater than (
>
) - ✅ Greater than or equal to (
>=
) - ✅ Less than (
<
) - ✅ Less than or equal to (
<=
)
- ✅ Greater than (
- ✅ Equality and logical negation expressions using
number
,boolean
,text
(string), andnone
can be evaluated.- ✅ Equal to (
=
) - ✅ Not equal to (
!=
) - ✅ Logical not (
not
)
- ✅ Equal to (
- ✅ Concatenation of
text
literals using+
can be evaluated.
Milestone 2: Support variables and control flow
-
✅ Temporary
@out
statement can be executed. -
✅ Global and local variables can be defined and used.
- ✅ Declaration and initialization (
var <name> : <expression>
) - ✅ Assignment expression (
<name> : <expression>
)
- ✅ Declaration and initialization (
-
✅ Variables adhere to lexical scope.
- ✅ Standalone block
block <statements> end
-
✅ Logical operators can be evaluated.
- ✅ Conjunction (
and
) - ✅ Disjunction (
or
)
- ✅ Conjunction (
-
⭕ Control flow statements can be executed.
- ⭕ Selection
- ✅
if
- ⭕
elseif
- ✅
else
- ✅
if <condition> <statements> elseif <condition> <statements> else <statements> end
- ✅ Loops
- ✅ Bounded (
foreach
)
- ✅ Bounded (
foreach <name> in <start>..<end> step <change> <statements> end
Example 1
// `<change>` expression is implicitly 1 foreach value in 0..10 @out value end
Example 2
var a: 0 var b: 10 var c: 2 foreach value in a..b step c @out value end
- ✅ Unbounded (
while
)
while <condition> { <change> } <statements> end
Example
// `<change>` expression is optional var i: 0 while i < 10 {i +: 1} @out i end
- ⭕ Selection
-
⭕ Augmented assignment expressions can be evaluated.
- ✅ Addition and assignment (
+:
) - ✅ Subtraction and assignment (
-:
) - ✅ Multiplication and assignment (
*:
) - ⭕ Division and assignment (
/:
) - ⭕ Modulo and assignment (
mod:
)
- ✅ Addition and assignment (
-
⭕ Range comparison expression can be evaluated (
<value> in <min>..<max>
)
More milestones will be added.
Milestone 3: Provide an interactive playground
- ✅ A Thusly VM can run and execute code in the browser via an interactive playground.
Milestone 4: Support first-class functions
- ⭕ Functions can be defined and invoked.
- ⭕ Closures are supported.
- ⭕ Functions are first-class citizens.
More milestones will be added.
See the source code README file for the most recent implementation.